シーンを自動で「Scenes In Build」に追加・削除する方法
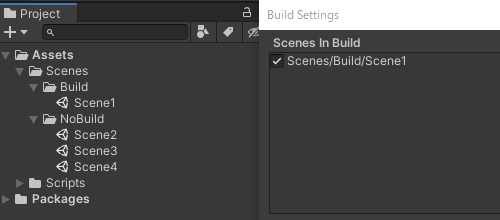
この記事では、Unityでシーンを自動で「Scenes In Build」に追加・削除するエディタ拡張の作り方について説明します。
作り方
AssetPostprocessorクラスを継承する
AssetPostprocessor
クラスを継承したクラスを作ります。
using UnityEditor;
public sealed class ScenePostprocessor : AssetPostprocessor
{
}
定数を宣言する
関数の実装に必要な定数を宣言します。
private const string BUILD_DIRECTORY_PATH = "Assets/Scenes/Build";
private const string SCENE_FILE_EXTENSION = ".unity";
シーンを追加・削除する関数を定義する
ここで新たなusing
ディレクティブが必要となるため、下記のコードを一番上の行に追加します。
using System.Linq;
using System.Collections.Generic;
そして、シーンを追加・削除をする関数をそれぞれ定義します。
シーンを追加する関数
private static void AddScenes(
List<EditorBuildSettingsScene> scene_list,
string[] imported_asset_paths,
string[] asset_paths_after_moving
) {
var adding_scenes = imported_asset_paths
.Concat ( asset_paths_after_moving )
.Where ( asset_path => asset_path.EndsWith( SCENE_FILE_EXTENSION ) )
.Where ( scene_file_path => scene_file_path.StartsWith( BUILD_DIRECTORY_PATH ) )
.Where ( scene_file_path => !scene_list.Any( scene => scene.path == scene_file_path ) )
.Select ( scene_file_path => new EditorBuildSettingsScene( scene_file_path, true ) )
;
scene_list.AddRange( adding_scenes );
}
シーンを削除する関数
private static void RemoveScenes(
List<EditorBuildSettingsScene> scene_list,
string[] deleted_asset_paths,
string[] asset_paths_after_moving
) {
var moved_scene_files_out_of_build = asset_paths_after_moving
.Where( asset_path => asset_path.EndsWith( SCENE_FILE_EXTENSION ) )
.Where( scene_file_path => !scene_file_path.StartsWith( BUILD_DIRECTORY_PATH ) )
;
scene_list.RemoveAll( scene => deleted_asset_paths.Contains( scene.path ) );
scene_list.RemoveAll( scene => moved_scene_files_out_of_build.Contains( scene.path ) );
}
OnPostprocessAllAssets関数を定義する
定義した関数を使用してOnPostprocessAllAssets
関数を定義します。これでエディタ拡張のプログラムは完成です。
private static void OnPostprocessAllAssets(
string[] imported_asset_paths,
string[] deleted_asset_paths,
string[] asset_paths_after_moving,
string[] asset_paths_before_moving
) {
var scene_list = EditorBuildSettings.scenes.ToList();
AddScenes ( scene_list, imported_asset_paths , asset_paths_after_moving );
RemoveScenes( scene_list, deleted_asset_paths , asset_paths_after_moving );
EditorBuildSettings.scenes = scene_list.ToArray();
}
使い方
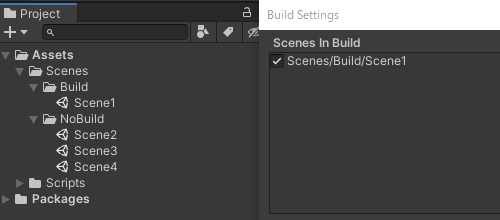
上記のプログラムを作成した後に「Assets/Scenes/Build
」( フォルダ )内でシーンを作成するか、そのフォルダ内にシーンを移動すると、「Scenes in Build」へシーンが追加されます。
また、「Assets/Scenes/Build
」内のシーンを削除する、あるいはそのフォルダ外へシーンを移動すると、「Scenes in Build」からシーンが削除されます。